UITableView is a basic UI element in iOS App. We can design a table view on the storyboard with its custom cells or XIB. But sometimes we need to create it at run time without a storyboard so we can assign specific frames and bounds to it. Creating UITableView programmatically in swift is a little complicated but it provides a better understanding of view hierarchy.
In this tutorial, we are going to talk about how to create basic UITableView programmatically without a storyboard or XIB. We only use code to design it and use frames and bounds to design its cells.
Here’s is the video if you prefer video over text.
1. Create a New Swift Project
Open Xcode, Select App and click on next and give a proper name to the project and make sure to select the user interface should be Swift, and create the new project. Give the name according to your need.
2. Initialize & Setup UITableView
First, we need to initialize the UITableView and set its frames so it can show on the screen according to our frames. I am using self.view frames to add the tableView on the complete screen you can change the frames according to your need.
var tableView = UITableView()
We also need to create one setTableView() method to configure the table view properties. In this method, we will define frames, backgroundColor, and other configurations. Now we will add this table view on the self.view so it can show on the screen.
Register one TableView cell class in this method, so we can use this cell to show the data on the tableView. We will see how we design this cell in the next step, but we need to register this cell in this method.
func setTableView() {
tableView.frame = self.view.frame
tableView.delegate = self
tableView.dataSource = self
tableView.separatorColor = UIColor.clear
tableView.backgroundColor = colorLiteral(red: 0.9254902005, green: 0.2352941185, blue: 0.1019607857, alpha: 1)
self.view.addSubview(tableView)
tableView.register(CustomTableViewCell.self, forCellReuseIdentifier: "Cell")
}
Before proceeding further, We need to create the model and create a variable to store raw data in it. You can get the raw data from the project link which is at the bottom.
class UserModal {
var userImage: UIImage?
var name: String?
var age: String?
init(userImage: UIImage, name: String, age: String) {
self.userImage = userImage
self.name = name
self.age = age
}
}
var userArr = [UserModal]()
Now, add some data in this userArr array, we use this array to populate table view.
override func viewDidLoad() {
super.viewDidLoad()
setTableView()
self.title = "User List"
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Charlize Theron"), name: "Amber Heard", age: "32"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Emma Stone"), name: "Emma Stone", age: "30"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Natalie Portman"), name: "Natalie Portman", age: "37"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Emma Watson"), name: "Emma Watson", age: "28"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Amber Heard"), name: "Angelina Jolie", age: "43"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Jennifer Lawrence"), name: "Scarlett Johansson", age: "34"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Angelina Jolie"), name: "Jennifer Lawrence", age: "28"))
userArr.append(UserModal(userImage: imageLiteral(resourceName: "Scarlett Johansson"), name: "Charlize Theron", age: "43"))
}
3. Design UITableView Cell
Now we have to populate the UITableView with the data, but before populating we need to design the UITableView Cell. Designing the cell is a little complicated cause we are using frames and positions to design UI elements. We required some maths so we can find the correct position of the UI elements which we need to place.
So, take a new class CustomTableViewCell.swift which we have already registered in the setupTableView() method. This is the cell class that is used to show the data on the screen and you can change the UI elements according to your need.
First, we create the UI element objects in the cell class and define the position of each element using frames with respect to the cell and other elements. We are using lazy property to define the UI elements, so these UI elements will get the memory when they get first in use.
lazy var backView: UIView = {
let view = UIView(frame: CGRect(x: 10, y: 6, width: self.frame.width - 20, height: 110))
view.backgroundColor = UIColor.white
return view
}()
lazy var userImage: UIImageView = {
let userImage = UIImageView(frame: CGRect(x: 4, y: 4, width: 104, height: 104))
userImage.contentMode = .scaleAspectFill
return userImage
}()
lazy var namelbl: UILabel = {
let lbl = UILabel(frame: CGRect(x: 116, y: 8, width: backView.frame.width - 116, height: 30))
lbl.textAlignment = .left
lbl.font = UIFont.boldSystemFont(ofSize: 18)
return lbl
}()
lazy var agelbl: UILabel = {
let lbl = UILabel(frame: CGRect(x: 116, y: 42, width: backView.frame.width - 116, height: 30))
lbl.textAlignment = .left
return lbl
}()
After defining all the properties, we need to add all the elements on the cell.
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
addSubview(backView)
backView.addSubview(userImage)
backView.addSubview(namelbl)
backView.addSubview(agelbl)
}
We also need to define some properties to some of the elements, so it can look good. We need to make the user image in round shape, so we can configure these properties on layout subview method.
override func layoutSubviews() {
contentView.backgroundColor = UIColor.clear
backgroundColor = UIColor.clear
backView.layer.cornerRadius = 5
backView.clipsToBounds = true
userImage.layer.cornerRadius = 52
userImage.clipsToBounds = true
}
3. Populate UITableView with Data
Now we are all done, we only need to populate the UITableView with the data which we have added in the setup method.
extension ViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return userArr.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as? CustomTableViewCell else {fatalError("Unabel to create cell")}
cell.userImage.image = userArr[indexPath.row].userImage
cell.namelbl.text = userArr[indexPath.row].name
cell.agelbl.text = userArr[indexPath.row].age
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 120
}
}
Now we can run the project and check the complete functionality.
Download Resources for Create UITableView Programmatically in Swift
You can download the source code of Create UITableView Programmatically in Swift project from this Link.
I hope you like this tutorial and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????
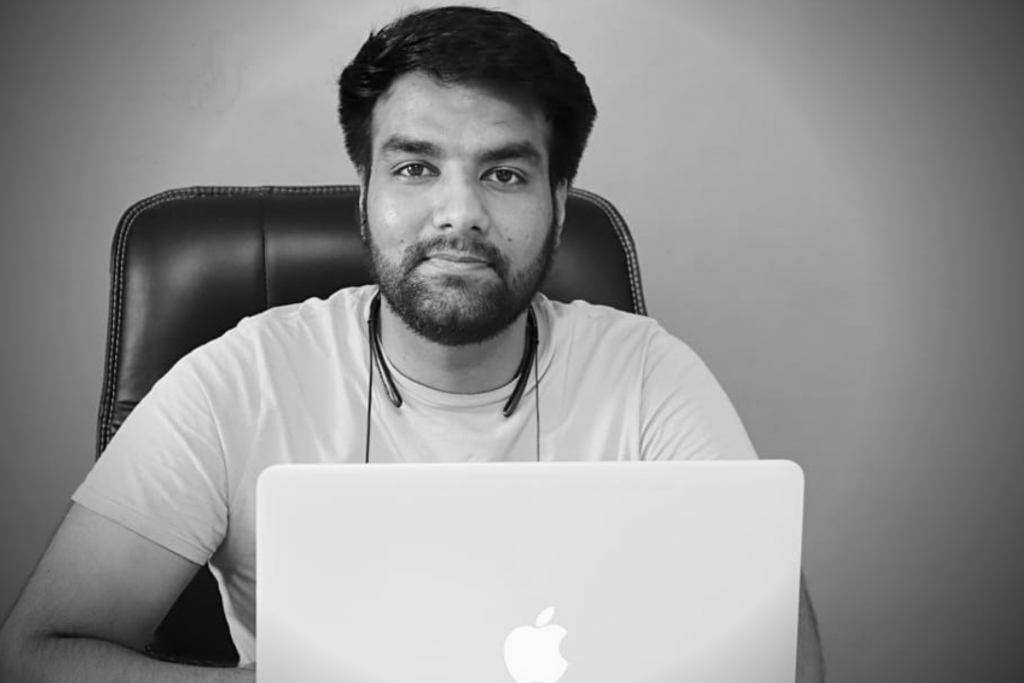
About the Author
Shubham Agarwal is a passionate and technical-driven professional with 5+ years of experience in multiple domains like Salesforce, and iOS Mobile Application Development. He also provides training in both domains, So if you looking for someone to provide you with a basic to advance understanding of any of the domains feel free to contact him