Searching is a very common feature in any application, Adding search to tableView in swift, allows us to search in the list of elements that are presenting on table view and it is very easy to implement. The search is case insensitive means you can search with capital or small letter it will find all the related matches.
Here’s is the video if you prefer video over text.
Note: This video is created with Xcode 9.0 and Swift 4.0, so maybe some functions are depreciated.
You should also have basic knowledge of UITableView.
1. Create a New Swift Project
Open Xcode, Select App and click on next and give a proper name to the project and make sure to select the user interface should be Swift, and create the new project.
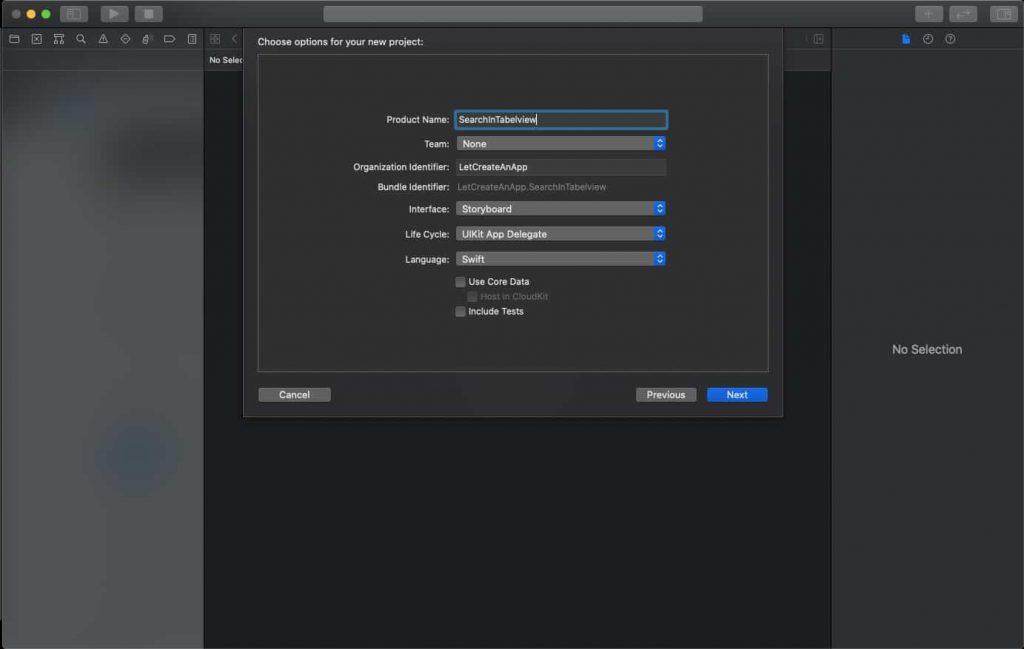
2. Add Search Bar and TableView
Let’s design the basic UI, in which we are having UISearchBar and UITableview. Add search bar at the top of the table view and give the basic constraint to search bar and table view. Add UITableViewCell in the table view and add one UILabel into it to show the country name in it. You can also change the design as per your need or you can follow the below design.
Also, confirm the data source and delegate of table and search bar, you can also confirm this in coding part. It is totally depend on you.
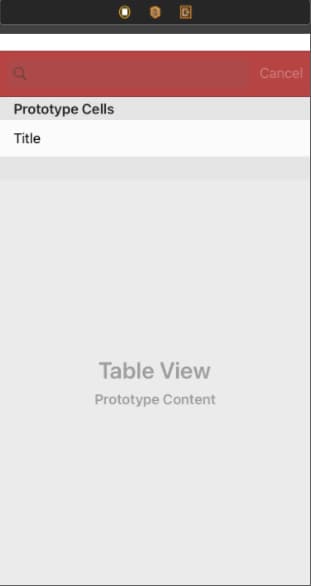
3. Setup TableView DataSource and Delegate
Now we need to load the country list into the tableview, so first we need to add the list of countries into the a variable and use that variable as the datasource for the tableview.
so, first add new variable which has list of all the countries.
let countryNameArr = ["Afghanistan", "Albania", "Algeria", "American Samoa", "Andorra", "Angola", "Anguilla", "Antarctica", "Antigua and Barbuda", "Argentina", "Armenia", "Aruba", "Australia", "Austria", "Azerbaijan", "Bahamas", "Bahrain", "Bangladesh", "Barbados", "Belarus", "Belgium", "Belize", "Benin", "Bermuda", "Bhutan", "Bolivia", "Bosnia and Herzegowina", "Botswana", "Bouvet Island", "Brazil", "British Indian Ocean Territory", "Brunei Darussalam", "Bulgaria", "Burkina Faso", "Burundi", "Cambodia", "Cameroon", "Canada", "Cape Verde", "Cayman Islands", "Central African Republic", "Chad", "Chile", "China", "Christmas Island", "Cocos (Keeling) Islands", "Colombia", "Comoros", "Congo", "Congo, the Democratic Republic of the", "Cook Islands", "Costa Rica", "Cote d'Ivoire", "Croatia (Hrvatska)", "Cuba", "Cyprus", "Czech Republic", "Denmark", "Djibouti", "Dominica", "Dominican Republic", "East Timor", "Ecuador", "Egypt", "El Salvador", "Equatorial Guinea", "Eritrea", "Estonia", "Ethiopia", "Falkland Islands (Malvinas)", "Faroe Islands", "Fiji", "Finland", "France", "France Metropolitan", "French Guiana", "French Polynesia", "French Southern Territories", "Gabon", "Gambia", "Georgia", "Germany", "Ghana", "Gibraltar", "Greece", "Greenland", "Grenada", "Guadeloupe", "Guam", "Guatemala", "Guinea", "Guinea-Bissau", "Guyana", "Haiti", "Heard and Mc Donald Islands", "Holy See (Vatican City State)", "Honduras", "Hong Kong", "Hungary", "Iceland", "India", "Indonesia", "Iran (Islamic Republic of)", "Iraq", "Ireland", "Israel", "Italy", "Jamaica", "Japan", "Jordan", "Kazakhstan", "Kenya", "Kiribati", "Korea, Democratic People's Republic of", "Korea, Republic of", "Kuwait", "Kyrgyzstan", "Lao, People's Democratic Republic", "Latvia", "Lebanon", "Lesotho", "Liberia", "Libyan Arab Jamahiriya", "Liechtenstein", "Lithuania", "Luxembourg", "Macau", "Macedonia, The Former Yugoslav Republic of", "Madagascar", "Malawi", "Malaysia", "Maldives", "Mali", "Malta", "Marshall Islands", "Martinique", "Mauritania", "Mauritius", "Mayotte", "Mexico", "Micronesia, Federated States of", "Moldova, Republic of", "Monaco", "Mongolia", "Montserrat", "Morocco", "Mozambique", "Myanmar", "Namibia", "Nauru", "Nepal", "Netherlands", "Netherlands Antilles", "New Caledonia", "New Zealand", "Nicaragua", "Niger", "Nigeria", "Niue", "Norfolk Island", "Northern Mariana Islands", "Norway", "Oman", "Pakistan", "Palau", "Panama", "Papua New Guinea", "Paraguay", "Peru", "Philippines", "Pitcairn", "Poland", "Portugal", "Puerto Rico", "Qatar", "Reunion", "Romania", "Russian Federation", "Rwanda", "Saint Kitts and Nevis", "Saint Lucia", "Saint Vincent and the Grenadines", "Samoa", "San Marino", "Sao Tome and Principe", "Saudi Arabia", "Senegal", "Seychelles", "Sierra Leone", "Singapore", "Slovakia (Slovak Republic)", "Slovenia", "Solomon Islands", "Somalia", "South Africa", "South Georgia and the South Sandwich Islands", "Spain", "Sri Lanka", "St. Helena", "St. Pierre and Miquelon", "Sudan", "Suriname", "Svalbard and Jan Mayen Islands", "Swaziland", "Sweden", "Switzerland", "Syrian Arab Republic", "Taiwan, Province of China", "Tajikistan", "Tanzania, United Republic of", "Thailand", "Togo", "Tokelau", "Tonga", "Trinidad and Tobago", "Tunisia", "Turkey", "Turkmenistan", "Turks and Caicos Islands", "Tuvalu", "Uganda", "Ukraine", "United Arab Emirates", "United Kingdom", "United States", "United States Minor Outlying Islands", "Uruguay", "Uzbekistan", "Vanuatu", "Venezuela", "Vietnam", "Virgin Islands (British)", "Virgin Islands (U.S.)", "Wallis and Futuna Islands", "Western Sahara", "Yemen", "Yugoslavia", "Zambia", "Zimbabwe"]
We also need one variable to hold the state of searching, its initial values will be false. If user is searching into the list that state will change and become true.
var isSearching = false
Add one more variable to hold the searched results. we will insert all those country in this array which will satisfy the user query, else we will show the complete list of country data.
var searchedCountry = [String]()
Now, We need to show this country data into the Tableview, so we need to integrate data sources and delegate methods to populate the table view.
First, create one extension to add the data source and delegate method of the table view. Now we have to confirm the protocol for this extension. Follow the below code to show the country list in a table view.
Note: Extension is always a good approach to write the delegate and data source methods. This increases the code readability and makes code manageable.
These are the methods which calls when data is loading into the table view.
extension ViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
if isSearching {
return searchedCountry.count
} else {
return countryNameArr.count
}
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell")
if isSearching {
cell?.textLabel?.text = searchedCountry[indexPath.row]
} else {
cell?.textLabel?.text = countryNameArr[indexPath.row]
}
return cell!
}
}
In the first method numberOfRowsInSection this calls to detect the number of elements to be load into the table view. We are loading data on the basis of isSearching variable. If isSearching is true means the user is searching into the table view, in that case, we need to load the from the searchedCountry this variable else we need to load the data on the basis of the original variable.
In a similar way, we are populating the table view cell label on the basis of isSearching variable, and showing the name of the country as per respective arrays, as you can see in the above code.
4. Setup SearchBar Delegate Methods.
Now, we have to add the search bar functionality and filter the array on the basis of the string entered by the user into the search bar. For getting the entered string into the search bar we need to add the delegate methods of the search bar. As we have already seen in the above section, how we have to add the delegate methods into the extension. We have to do the same thing for UISearchBar delegate methods.
extension ViewController: UISearchBarDelegate {
func searchBar(_ searchBar: UISearchBar, textDidChange searchText: String) {
searchedCountry = countryNameArr.filter({$0.lowercased().prefix(searchText.count) == searchText.lowercased()})
isSearching = true
tblView.reloadData()
}
func searchBarCancelButtonClicked(_ searchBar: UISearchBar) {
isSearching = false
searchBar.text = ""
tblView.reloadData()
}
}
UISearchBar delegate method textDidChange calls when users type anything in their search box. We are filtering the countryNameArr on the basis of prefix text which is entered by the user into the search box and we will get the new array with filtered data into searchedCountry variable and we also changing the state of isSearching variable to true, which means searching is enabled and loading the table view again with the filtered data(searchedCountry).
Now we can run the project and check the complete functionality.
Download Resources for Adding Search to TableView in Swift
You can download the source code of Adding Search to TableView in Swift project from this link.
I hope you like this tutorial and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????
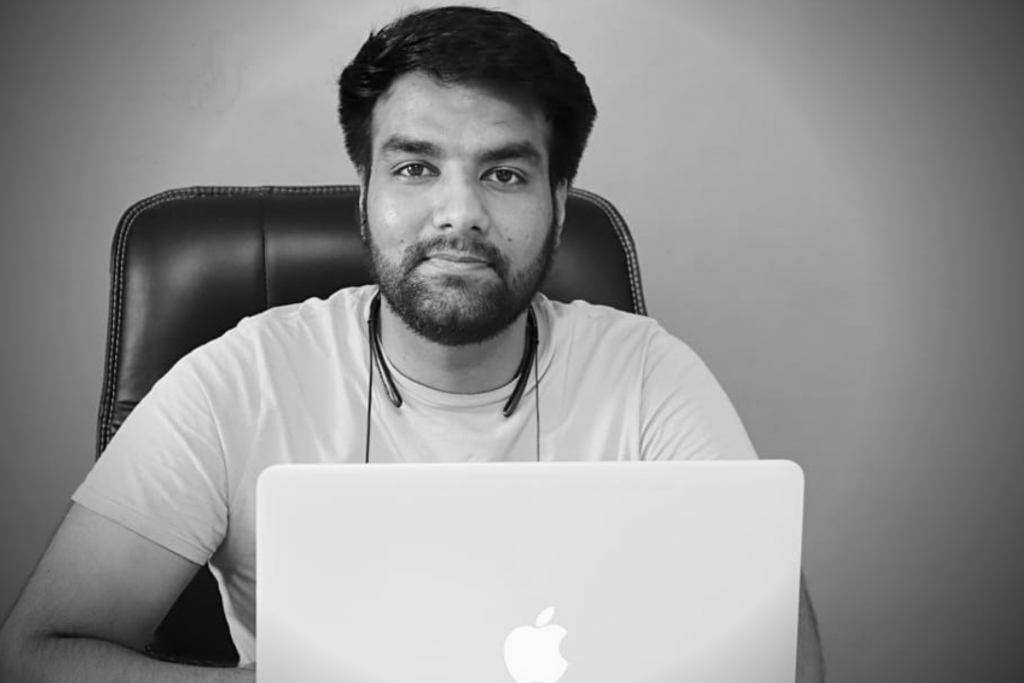
About the Author
Shubham Agarwal is a passionate and technical-driven professional with 5+ years of experience in multiple domains like Salesforce, and iOS Mobile Application Development. He also provides training in both domains, So if you looking for someone to provide you with a basic to advance understanding of any of the domains feel free to contact him
The search bar settings are complete.
Thanks from Japan.