Having a collection view inside table view has become a very common design pattern. Many of the applications are already using this kind of pattern in their apps like Spotify, App store, etc. Adding collectionView to tableView is not a complex functionality but managing actions and data is a little tricky.
This kind of functionality is useful when we have to show a vertical list (UITableView) with a horizontal list(UICollectionView). In which we have added collectionView inside tableView.
In this article, we will see how we can add collectionView inside tableView. If you want to take an action on the cells and want to move on some details screens, please read Add CollectionView inside TableView article to understand more about this functionality.
This is part 8 of AutoLayout and Constraint series, if you didn’t watch the previous video, please watch them.
Here’s is the video if you prefer video over text:
1. Add UITableView & UICollectionView on the Explore Tab
First, we need to move on the explore tab, and add the UITableView on it. After adding UITableView inside of explore tab, add UITableViewCell in the UITableView. UITableView cell will hold the UICollectionView and its functionality.
Add UICollectionView inside the UITableView cell and give the constraint to UICollectionView. Add one UICollectionView cell inside the UICollectionView. Design this cell as per your need, in this article I am taking one image and one label as you can see in the image below.
Now, if you see the hierarchy so it will look something like this UITableView -> UITableViewCell-> UICollectionView-> UICollectionViewCell. TableView is the parent of all the class, so all the code related to the data fetching or API calls will be written in TableView class.
With all the setup, the storyboard look something like below image.

2. Show CollectionView with Data.
After setup the design in the main.storyboard, you can create new ExploreViewController.swift class and take the outlet of tableview in that.
@IBOutlet weak var tableView: UITableView!
After taking the outlet we need to confirm the datasource and delegate of tableview and implement those methods.
extension ExploreViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 5
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) as? ExploreTableViewCell
return cell!
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
150
}
}
you can run the application check it, the tableview is start showing but UICollectionView is not visible. it is not showing because we didn’t setup UICollectionView till so far.
For setting up collectionView we need to work on UITableView cell class, so let’s move on ExploreTableViewCell class.
This class is responsible for handling the collectionView and its data. So take the outlet of collection view and its delegate method in this class.
@IBOutlet weak var collectionView: UICollectionView!
override function awakeFromNib() {
super.awakeFromNib()
collectionView.delegate = self
collectionView.dataSource = self
}
Finally we need to provide the datasource and delegate methods in UITableView cell class. So when class get load it will also load the UICollectionView.
extension ExploreTableViewCell: UICollectionViewDelegate, UICollectionViewDataSource {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 7
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "ProductCollectionViewCell", for: indexPath)
return cell
}
}
Now you can run the application and test the functionality. However if you face difficulty in this article please watch video tutorial with complete explanations.
Download Resources
You can download the source code of Adding UICollectionView to UITableView Cell project from this Github link.
I hope you like this tutorial and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????
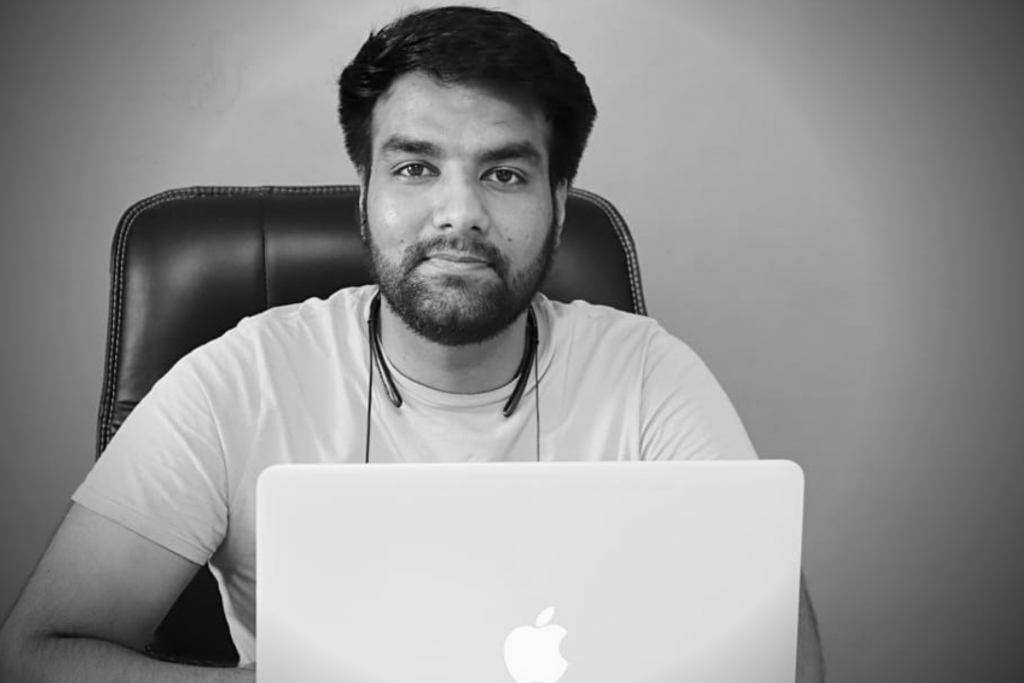
About the Author
Shubham Agarwal is a passionate and technical-driven professional with 5+ years of experience in multiple domains like Salesforce, and iOS Mobile Application Development. He also provides training in both domains, So if you looking for someone to provide you with a basic to advance understanding of any of the domains feel free to contact him