Viewing images closely by pinch to zoom them is very common in any iOS app. ImageView is used to show the images but unfortunately, ImageView doesn’t come up with this functionality. So we need to programmatically create a pinch to zoom image In/out functionality.
In this tutorial, we will see how we can zoom the image by pinching on the imageView and how we manage the empty space issues that occur when we zoom the image.
Here’s is the video if you prefer video over text.
1. Create a New Swift Project
Open Xcode, Select App and click on next and give a proper name to the project and make sure to select the user interface should be Swift, and create the new project. Give the name according to your need.
2. Create Basic Design & Outlets
For designing, we required one UIScrollView and UIImageView. We need to first put the UIScrollView on parent view and give the constraints all the four sides to 0, this will place the UIScrollView on the screen. Now take and UIImageView and put the image view on the top of UIScrollView and give all the four sides to 0 and also provide equal width and equal height with respect to the parent view.
Add any image into the asset folder and set that image on the ImageView. Make sure aspect mode of that image should be aspect fit.
If you want to learn more about how to add UIScrollView on storyboard, please watch this.
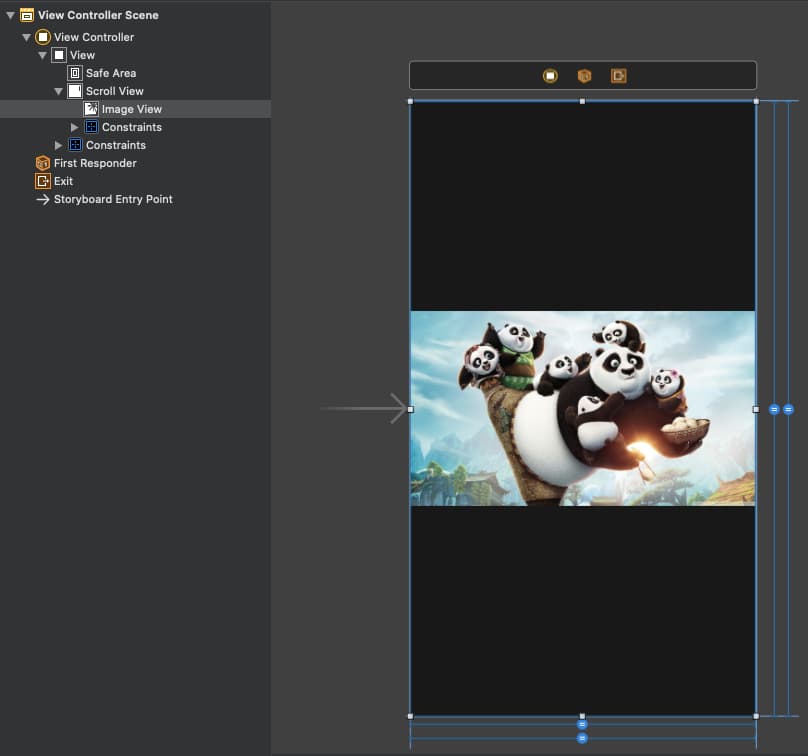
Now, Take the outlet of UI elements on the ViewController.swift class and provide some basic properties to scroll view.
@IBOutlet weak var scrollView: UIScrollView!
@IBOutlet weak var imageView: UIImageView!
Configure some UIScrollView properties, you can change these properties according to your need and requirement. I am using standard values for these properties.
override func viewDidLoad() {
super.viewDidLoad()
scrollView.maximumZoomScale = 4
scrollView.minimumZoomScale = 1
scrollView.delegate = self
}
ScrollView zoom scale properties use to define number of time images can be zoom. We can use any number but try to make it relevant otherwise image get blur and faded.
3. Use UIScrollView Delegate to Zoom Image In/Out
UIScrollView provide us some delegate functionality by using that we can zoom the content in its own bounds. So for that first we need to confirm the Protocol.
extension ViewController: UIScrollViewDelegate {
}
Now need to confirm delegate methods into the above block of code, which allows us to make the image zoom and maintain the aspect ratio.
There are 2 delegates methods which we need to use.
- func viewForZooming(in scrollView: UIScrollView) -> UIView?
- func scrollViewDidZoom(_ scrollView: UIScrollView)
- viewForZooming delegate function is used to return the view on which we need to zoom, in our case it is UIImageView. You can also use UIView if you are using some other things to zoom.
extension ViewController: UIScrollViewDelegate {
func viewForZooming(in scrollView: UIScrollView) -> UIView? {
return imageView
}
- scrollViewDidZoom delegate function is used to zoom the image, by zooming the image we will come across one issue in which a zoomed image will leave empty spaces at the edges because imageView has content mode as aspect fit. To overcome this issue we are writing some logic that maintains the aspect ratio even if a user is zooming the image.
func scrollViewDidZoom(_ scrollView: UIScrollView) {
if scrollView.zoomScale > 1 {
if let image = imageView.image {
let ratioW = imageView.frame.width / image.size.width
let ratioH = imageView.frame.height / image.size.height
let ratio = ratioW < ratioH ? ratioW : ratioH
let newWidth = image.size.width * ratio
let newHeight = image.size.height * ratio
let conditionLeft = newWidth*scrollView.zoomScale > imageView.frame.width
let left = 0.5 * (conditionLeft ? newWidth - imageView.frame.width : (scrollView.frame.width - scrollView.contentSize.width))
let conditioTop = newHeight*scrollView.zoomScale > imageView.frame.height
let top = 0.5 * (conditioTop ? newHeight - imageView.frame.height : (scrollView.frame.height - scrollView.contentSize.height))
scrollView.contentInset = UIEdgeInsets(top: top, left: left, bottom: top, right: left)
}
} else {
scrollView.contentInset = .zero
}
}
Now we can run the project and check the complete functionality.
Download Resources for Pinch to Zoom image In/Out Programmatically
You can download the source code of Pinch to Zoom image In/Out Programmatically project from this Link.
I hope you like this tutorial and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????
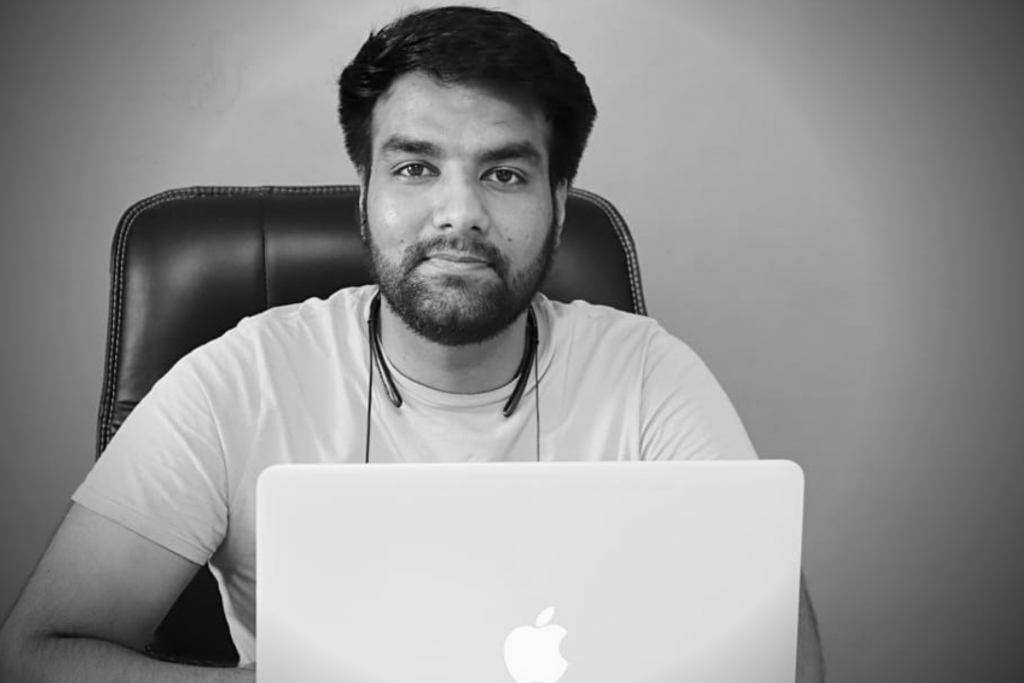
About the Author
Shubham Agarwal is a passionate and technical-driven professional with 5+ years of experience in multiple domains like Salesforce, and iOS Mobile Application Development. He also provides training in both domains, So if you looking for someone to provide you with a basic to advance understanding of any of the domains feel free to contact him
How we can manage imageview 90 degree rotation with zoom…
Thank you for this article! Very helpful!
For Xamarin devs, here is my adaptation: https://gist.github.com/DmitriyKirakosyan/c6b212f36b9c038a9d32d66eeaeedddc.