Navigation Bar is the most common component in the iOS Apps, it allows us to navigate the application. As we all know there is NO back button on the iPhones. So navigation bar allows us to move back from any controller by giving the back the button option by default. Add Button on Navigation Bar in Swift 5 is very easy and has basic functionality.
Sometimes, we come across the functionality in which we can have a requirement to add buttons on the navigation bar. So in this tutorial, we are going to cover how we can add buttons to the navigation bar in swift 5. We can add a button on the navigation bar in possibly 2 ways. I will try to make you clear both ways.
Two ways to add a button on the navigation bar
- Add a button directly from the storyboard.
- Add using code.
1. Add a button directly from the storyboard
This is the first and easy approach in which we are using a storyboard. Putting components on view using storyboard is very easy, We only need to drag and drop the component on the view controller.
So move on to the storyboard and search for UIBarButtonItem on the component library. And drag and drop that button on the Navigation bar as you can see this on the below image.
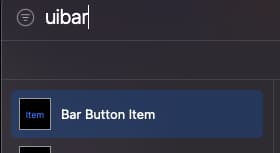
Now drag and drop this Bar Button Item on the Navigation Controller on top of ViewController. You can add multiple
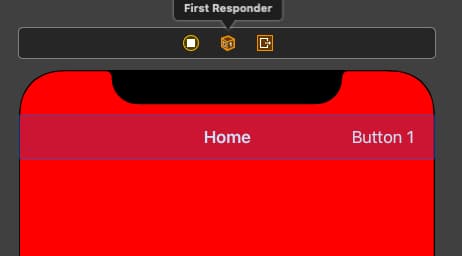
Now move on to the ViewController and create the action of this button. You need simply drag from this button to view the controller as we do for the simple button.
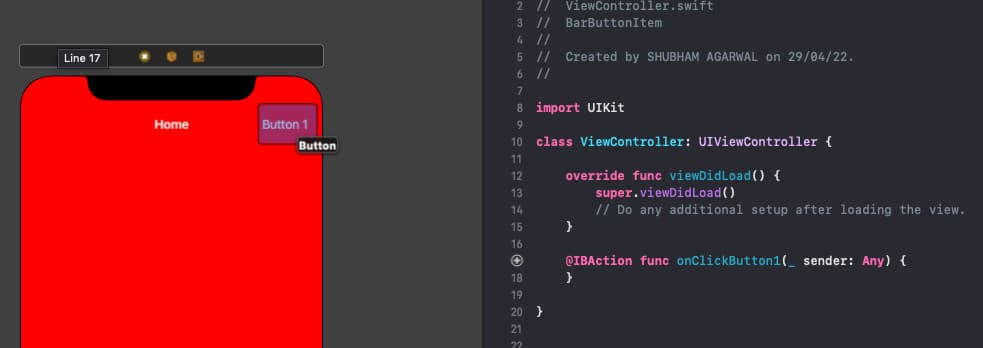
You can add more buttons in a similar way, and add action directly on the view controller.
2. Add a button on the navigation bar by coding
In this approach, we will use code to add a button on the navigation bar and create action on the controller. This approach is more useful when we need to add or remove buttons on navigation bar conditional bases.
So, first, we need to create the variable of UIBarButtonItem. We will get multiple overloaded methods with this button so we can choose one of them. We can select an image as a button or a title as a button. In this tutorial, we are using an image as a button.
We need to create a variable of an image or can directly assign an image literal to this method.
var messageImage = UIImage(named: "message")
messageImage = messageImage?.withRenderingMode(.alwaysOriginal)
Now, we need to assign this image to UIBarButtonItem, So it can show on the Navigation bar.
self.navigationItem.rightBarButtonItems = [UIBarButtonItem(image: messageImage, style:.plain, target: self, action: #selector(onClickMessageButton)]
@objc func onClickMessageButton() {
print("Message")
}
For creating the action of this button, We need to provide the selector to this UIBarButtonItem as we have provided in the above example.
We can also assign multiple buttons to the navigation controller by simply, assigning the array of buttons to this property.
self.navigationItem.rightBarButtonItems = [UIBarButtonItem(image: messageImage, style:.plain, target: self, action: #selector(onClickMessageButton)),
UIBarButtonItem(image:notificationImage, style:.plain, target: self, action: #selector(onClickNotificationButton))]
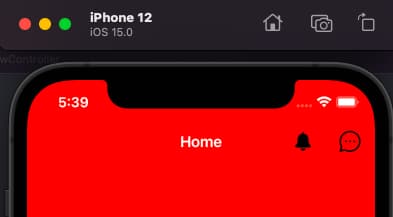
Conclusion
These are some simple ways, by which we can add a button on the navigation bar in swift 5.
I hope you like this tutorial and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????
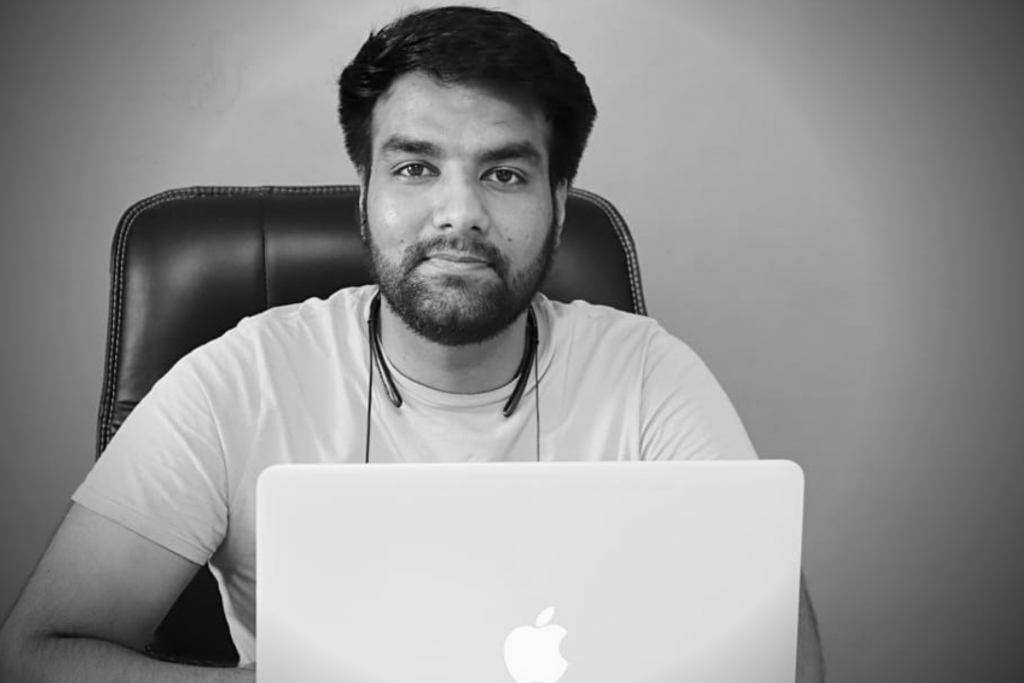
About the Author
Shubham Agarwal is a passionate and technical-driven professional with 5+ years of experience in multiple domains like Salesforce, and iOS Mobile Application Development. He also provides training in both domains, So if you looking for someone to provide you with a basic to advance understanding of any of the domains feel free to contact him