Singleton class in swift is a very useful and popular design pattern in development. Most of the developers are using this design pattern. This is very simple, common, and easy to use in your project. Singleton class helps you to share the same instance of variable in the complete application.
It initializes your class instance single time only with a static property. And it will share your class instance globally among all the controllers and swift files.
Singleton class can be used in any kind of Architecture pattern like MVC, MVVM.
What is Singleton class?
The singleton classes pattern guarantees that only one instance of a class is instantiated. That instance will be used in the complete project, No new instance will create. That’s simple. Right?
In simple words, we can say that the memory address of the singleton instance remains the same throughout the application session. So if anything is stored in any variable of this class can fetch from anywhere in the applications.
We have used so many Apple APIs which work as a singleton class like:
// Shared URL Session
let sharedURLSession = URLSession.shared
// Standard User Defaults
let standardUserDefaults = UserDefaults.standard
The singleton pattern is a very useful pattern. There are times that you want to make sure only one instance of a class is instantiated. So that your application only uses that instance. That’s the primary and only goal of the singleton pattern.
How to Create a Singleton In Swift
So for creating the Singleton class, We need to take a swift class and Initialize a static variable in that class of that same class type. Let’s create one String property in this class, So we can save the data and fetch it again. You can see that in the below example.
import Foundation
class SingleTon {
static let instance = SingleTone()
var savedText = ""
}
Let’s Use This Class
We can directly fetch the instance variable by the class name. We can create properties in the class to store the values in those properties. Let’s save some text in the savedText property.
SingleTone.instance.savedText = "Some Text is Saved"
By executing the above code, Sample text will store in the savedText property. Now let’s see how we can fetch the property back from singleTon class.
var saveProperty = SingleTone.instance.savedText
The data which is stored in the savedText property will be fetched and stored into the saveProperty variable.
You can store any kind of property in SingleTon class and fetch in the same way.
If you check the memory location of singleton variables in different classes, they will come up the same, check the below images and code.
print("\(SingleTone.instance.savedText.hashValue) First View Controller")
print("\(SingleTone.instance.savedText.hashValue) Second View Controller")

The memory location of the singleton variable is always the same in the complete application.
Better Way to Write Singelton Classes
In the above code, we have some loopholes, by which anyone can alter the singleton class and this is not a good option. So we need to put 2 points into consideration while writing singleton classes.
- Singelton class should not be Extendable.
- Singelton classes should not be initiate outside the class itself.
So, To overcome this problem, We need to make the class Final and make the default initializer private. You can see the code in the
below example.
import Foundation
final class SingleTone {
static let instance = SingleTone()
private init() { }
var savedText = ""
}
So, Now singleton classes can not be initialized in other classes and can not be extended.
I hope you like this tutorial on Singleton class in Swift and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????
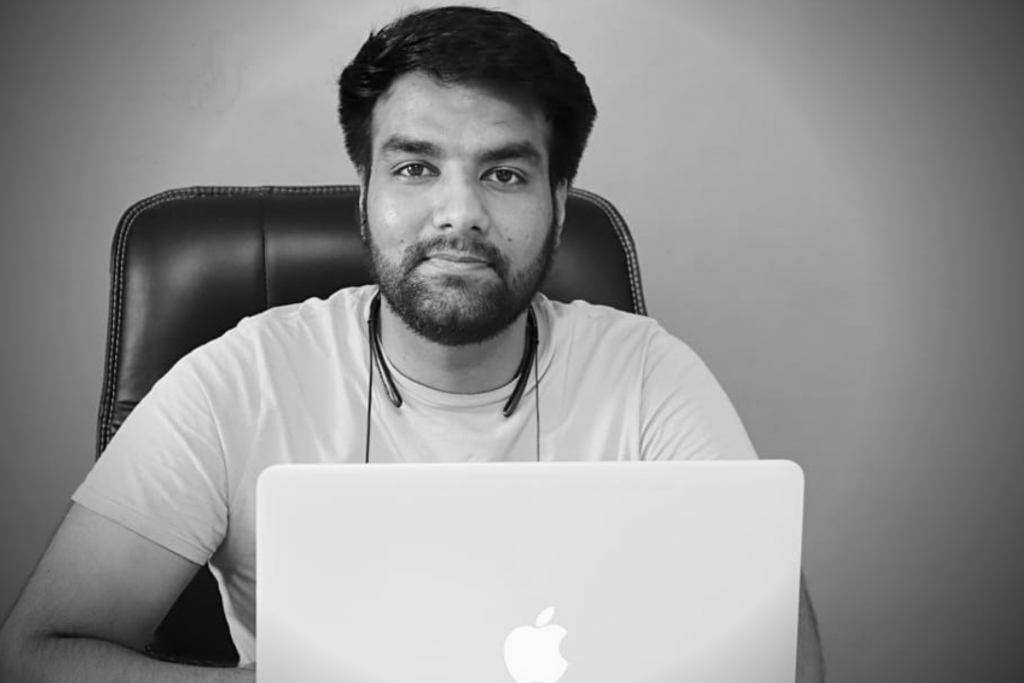
About the Author
Shubham Agarwal is a passionate and technical-driven professional with 5+ years of experience in multiple domains like Salesforce, and iOS Mobile Application Development. He also provides training in both domains, So if you looking for someone to provide you with a basic to advance understanding of any of the domains feel free to contact him
Pingback: MVVM in Swift - Let Create An App