Auto image slider is wrapping up multiple images in horizontal UICollectionView and then scroll those images automatically. It same as we can see on webpages. Image slider can be more common and useful than you may think.
Here’s is the video if you prefer video over text.
Final Output:
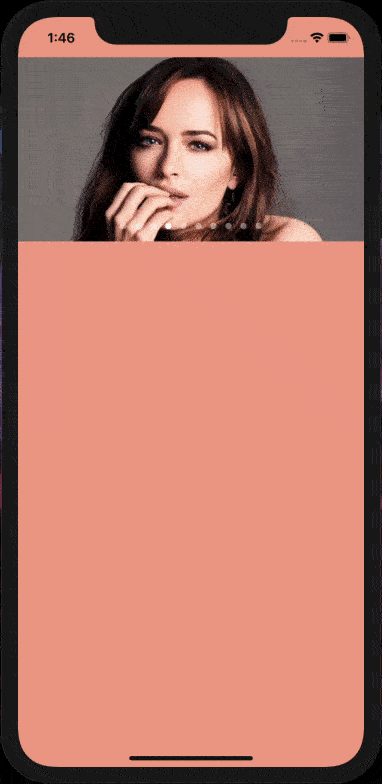
Prerequisite:
You must have knowledge of how CollectionView and its Delegate work, if not then watch this tutorial first then come back on this.
1. Create a New Swift Project
Open Xcode, Select Single View Application and click on next and give a proper name to the project and make sure to select the user interface should be Swift, and create the project.
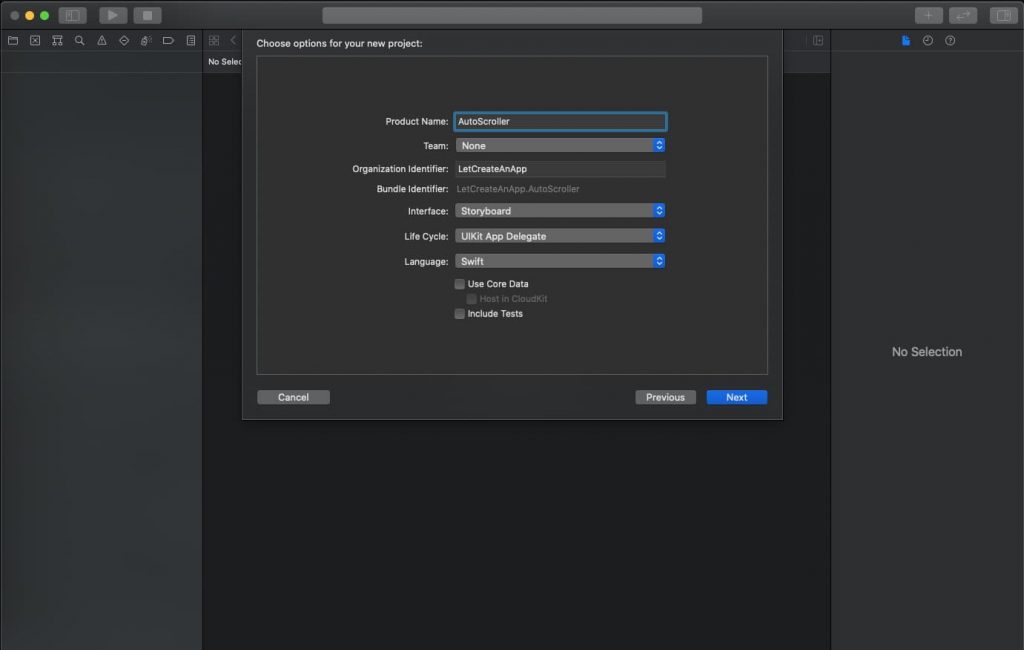
2. Create Basic UI
First, we have to take one UICollectionView and drop it on the UIViewController on the storyboard and we also need to drop the page controller to show dots at the bottom of the images. Now give some basic constraints to UICollectionView and PageController.
Now, We need to design the UICollectionView cell to show the images in it. After the complete design the UIViewController look something like this.
Note: Page controller will not be the part of UICollectionView Cell, It is the part of UIViewController.
Note: UICollectionView scrolling direction should be Horizontal.
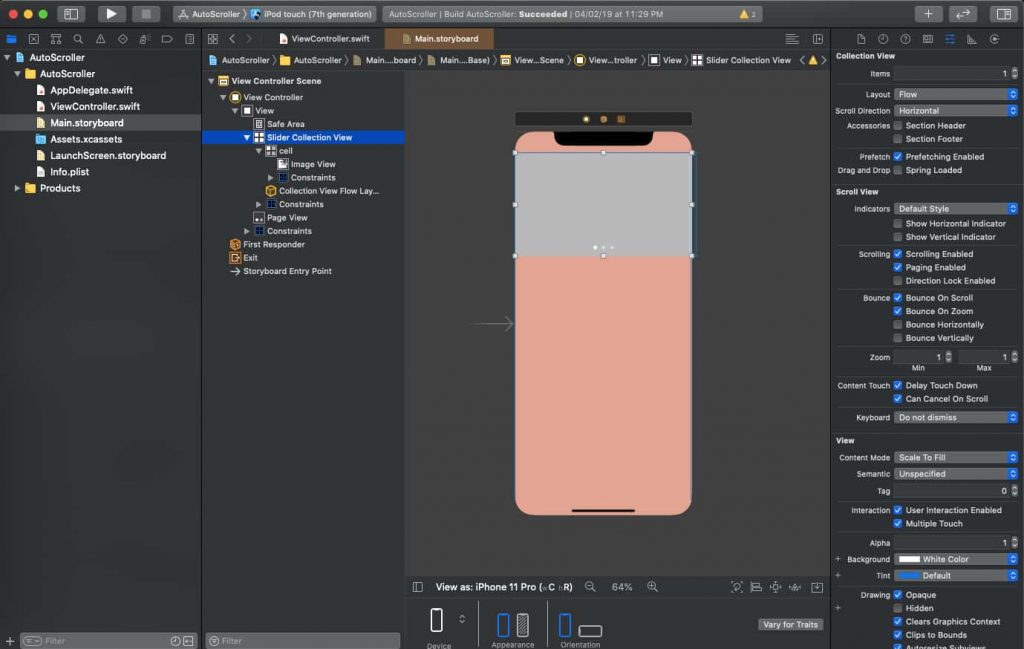
2. Setup UICollectionView and Delegate methods
Take the outlets of UICollectionView and PageController.
@IBOutlet weak var sliderCollectionView: UICollectionView!
@IBOutlet weak var pageView: UIPageControl!
Now, Setup the collection view as we have learned in the video Tutorials or you can download the project from the link below to get the setup.
After successful set the output will look something like this.
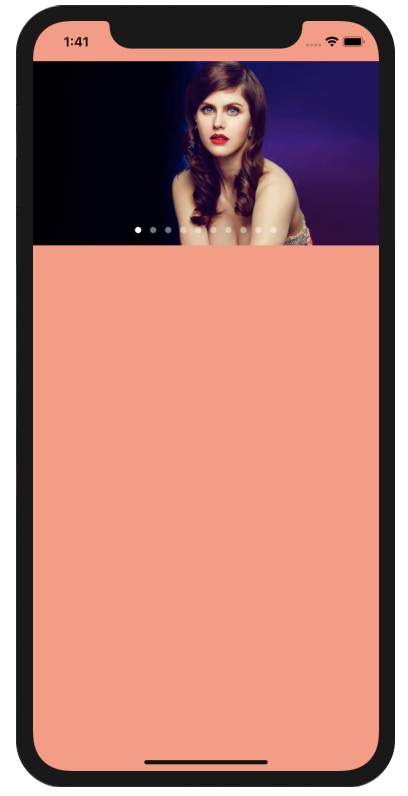
3. Auto Image Slider/Scroller Setup.
Now we need to write code to make the images auto slide in a specific period of time, for that we need to define one Timer and Counter variable to maintain the counts.
var timer = Timer()
var counter = 0
In viewDidLoad we need to define the Timer and configure the Page Controller as per our requirement, we are configuring the timer with 1 second and repeat to true, so it will call changeImage method every second and scroll to the next image automatically.
pageView.numberOfPages = imgArr.count
pageView.currentPage = 0
DispatchQueue.main.async {
self.timer = Timer.scheduledTimer(timeInterval: 1.0, target: self, selector: #selector(self.changeImage), userInfo: nil, repeats: true)
}
In changeImage method, we updating the counter variable value, so if the counter will reach the end of the image array, it can start over again.
sliderCollectionView.scrollToItem is use to scroll the UICollectionView to the specific cell. We only need to pass the IndexPath on which we want to scroll, So we will convert the counter as IndexPath and pass it to this method.
@objc func changeImage() {
if counter < imgArr.count {
let index = IndexPath.init(item: counter, section: 0)
self.sliderCollectionView.scrollToItem(at: index, at: .centeredHorizontally, animated: true)
pageView.currentPage = counter
counter += 1
} else {
counter = 0
let index = IndexPath.init(item: counter, section: 0)
self.sliderCollectionView.scrollToItem(at: index, at: .centeredHorizontally, animated: false)
pageView.currentPage = counter
counter = 1
}
}
Now build the complete code and run the application to see the Auto image slider/scroller works.
You can download the source code of this project from this this link.
I hope you like this tutorial and if you want any help let me know in the comment section.
Stay tuned, there is way more to come! follow me on Youtube, Instagram, Twitter. So you don’t miss out on all future Articles and Video tutorials.
. . .
I am delighted that you read my article! If you have any suggestions do let me know! I’d love to hear from you. ????